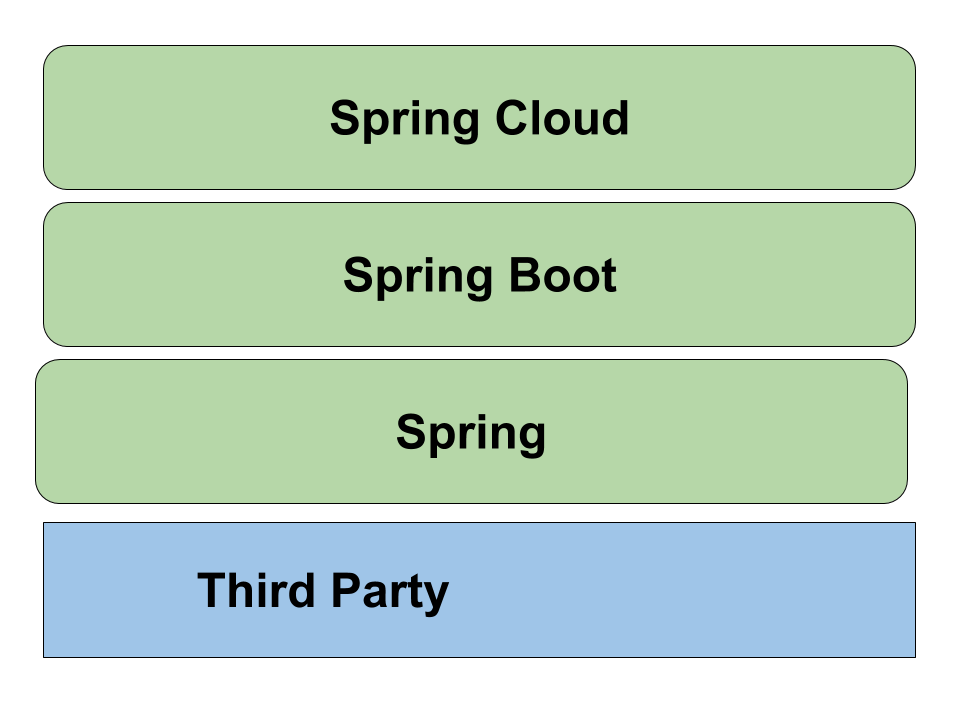
November 2017:
try {
Person p = personRepository.findById(id);
handle(p);
} catch (IOException ex) {
error(ex);
}
interface PersonRepository {
Person findById(String id);
List<Person> findAll();
void save(Person person);
}
Mono<Person> p = personRepository.findById(id)
.map(this::handle)
.doOnError(this::error);
interface PersonRepository {
Mono<Person> findById(String id);
Flux<Person> findAll();
Mono<Void> save(Person person);
}
@GetMapping("/users/{id}")
User getById(@PathVariable String id) {
return this.userRepository.findOne(id);
}
@GetMapping("/users/{id}")
Mono<User> getById(@PathVariable String id) {
return this.userRepository.findOne(id);
}
@Bean
public RouterFunction<?> userEndpoints() {
return route(GET("/users/{id}"), request ->
ok().body(
repository.findOne(request.pathVariable("id")),
User.class
));
}
WebClient client = WebClient.create();
Mono<GithubUser> githubUser = client
.get()
.uri("https://api.github.com/users/{username}", username)
.retrieve()
.bodyToMono(GithubUser.class);
Mono<TwitterUser> twitterUser = client
.get()
.uri("https://api.twitter.com/1.1/users/show.json?screen_name={username}", username)
.retrieve()
.bodyToMono(TwitterUser.class);
return githubUser.and(twitterUser,
(github, twitter)-> new AppUser(github, twitter));
client.get().uri("/users/dave")
.exchange()
.expectBody(User.class)
.consumeWith(result ->
assertThat(result.getResponseBody().getName())
.isEqualTo("dave"));
}
N.B. with @SpringBootTest
just @AutoConfigureWebTestClient
and
@Autowired
it.
More for scalability and stability than for speed
Servlets and Web MVC are not going to go away.
Project | Release | Timeline |
---|---|---|
Reactor |
Bismuth |
|
Framework |
5.0.2.RELEASE |
2017Q4 |
Data |
Kay |
|
Security |
5.0.0.RELEASE |
|
Integration |
5.0.0.RELEASE |
|
… |
|
|
Boot |
2.0.0.RELEASE |
2018Q1 |
Cloud |
Finchley.RELEASE |
|
Finchley: 2018Q1: Spring Boot 2.0.x
/