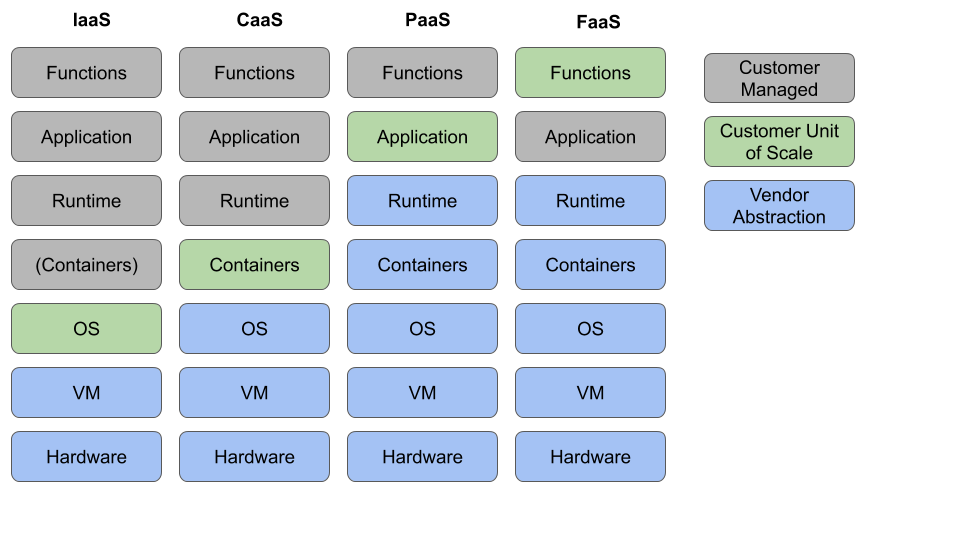
Credit: Olivier Tardieu, and John McKim
Credit: Olivier Tardieu, and John McKim
CNCF WG Whitepaper
Credit: Yan Cui, https://theburningmonk.com
(J) = native Java support
Others can run Java, e.g. via custom container or node JRE launcher.
public interface Function<T, R> {
R apply(T t);
}
public interface Consumer<T> {
void accept(T t);
}
public interface Supplier<T> {
T get();
}
@SpringBootApplication
public class Application {
@Bean
public Function<String, String> uppercase() {
return value -> value.toUpperCase();
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
package functions;
public class Uppercase implements Function<String, String> {
String apply(String input) {
return input.toUpperCase();
}
}
All the benefits of serverless, but with full access to Spring (dependency injection, integrations, autoconfiguration) and build tools (testing, continuous delivery, run locally)
For Spring devs: a smaller, more familiar step than using FaaS APIs and UIs natively
For Functionistas: no need to know anything about Spring
Decouple lifecycle of business logic from runtime platform. Run the same code as a web endpoint, a stream processor, or a task
Uniform programming model across serverless providers, and also able to run standalone (locally or in a PaaS)
public abstract class Flux<T> implements Publisher<T> {
...
}
public abstract class Mono<T> implements Publisher<T> {
...
}
@SpringBootApplication
public class Application {
@Bean
public Function<Flux<String>, Flux<String>> uppercase() {
return flux -> flux
.filter(this::isNotRude)
.map(String::toUpperCase);
}
boolean isNotRude(String word) {
...
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
@Beans
of type Function
, Consumer
and Supplier
, with Flux
, Mono
, Publisher
/